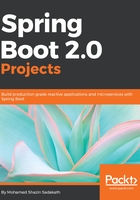
Anatomy of a Spring Boot application
The anatomy of a Spring Boot application will be that it will be inheriting from a spring-boot-starter-parent project that will in return have all the common dependencies available for a Spring Boot application. Apart from this, there will be one or more spring-boot-starter POM such as spring-boot-starter-web, spring-boot-starter-jpa, and so on. The following example excerpt from pom.xml shows the basic dependencies of a Spring Boot application:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
...
</dependencies>
The minimum bootstrapping point of a Spring Boot application will be a class with a main method that will be annotated with a @SpringBootApplication annotation along with the main method body, which calls the SpringApplication.run static method, for which a configuration class (a class with @Configuration annotation—the @SpringBootApplication annotation transitively has one) needs to be passed, along with a String array of arguments. The following code shows the minimum bootstrapping point of a Spring Boot application:
import org.springframework.boot.ApplicationRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class SpringBootIntroApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootIntroApplication.class, args);
}
@Bean
public ApplicationRunner applicationRunner() {
return args -> {
System.out.println("Hello, World!");
};
}
}
By running the preceding class, a Spring Boot application can be provisioned and executed. There are several ways to run a Spring Boot application; some of them are mentioned here:
- Running the Spring Boot application main class using an IDE.
- Building a JAR or WAR file using the following Maven command and then running:
$ mvn clean install
$ java -jar target/<package-name>.[jar|war]
- Run this using the Spring Boot Maven plugin:
$ mvn clean spring-boot:run
The @SpringBootApplication annotation comprises of @EnableAutoConfiguration and @ComponentScan annotations that do the heavy lifting of auto-configuring the Spring Boot application with the default settings and scanning the packages for any Spring-specific components such as services, components, repositories, and so on.