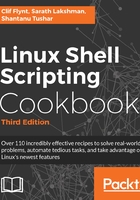
How to do it...
The echo command is the simplest command for printing in the terminal.
By default, echo adds a newline at the end of every echo invocation:
$ echo "Welcome to Bash" Welcome to Bash
Simply, using double-quoted text with the echo command prints the text in the terminal. Similarly, text without double quotes also gives the same output:
$ echo Welcome to Bash Welcome to Bash
Another way to do the same task is with single quotes:
$ echo 'text in quotes'
These methods appear similar, but each has a specific purpose and side effects. Double quotes allow the shell to interpret special characters within the string. Single quotes disable this interpretation.
Consider the following command:
$ echo "cannot include exclamation - ! within double quotes"
This returns the following output:
bash: !: event not found error
If you need to print special characters such as !, you must either not use any quotes, use single quotes, or escape the special characters with a backslash (\):
$ echo Hello world !
Alternatively, use this:
$ echo 'Hello world !'
Alternatively, it can be used like this:
$ echo "Hello World\!" #Escape character \ prefixed.
When using echo without quotes, we cannot use a semicolon, as a semicolon is the delimiter between commands in the Bash shell:
echo hello; hello
From the preceding line, Bash takes echo hello as one command and the second hello as the second command.
Variable substitution, which is discussed in the next recipe, will not work within single quotes.
Another command for printing in the terminal is printf. It uses the same arguments as the C library printf function. Consider this example:
$ printf "Hello world"
The printf command takes quoted text or arguments delimited by spaces. It supports formatted strings. The format string specifies string width, left or right alignment, and so on. By default, printf does not append a newline. We have to specify a newline when required, as shown in the following script:
#!/bin/bash #Filename: printf.sh printf "%-5s %-10s %-4s\n" No Name Mark printf "%-5s %-10s %-4.2f\n" 1 Sarath 80.3456 printf "%-5s %-10s %-4.2f\n" 2 James 90.9989 printf "%-5s %-10s %-4.2f\n" 3 Jeff 77.564
We will receive the following formatted output:
No Name Mark 1 Sarath 80.35 2 James 91.00 3 Jeff 77.56